How to Refactor Your Rails App With Service Objects
Blog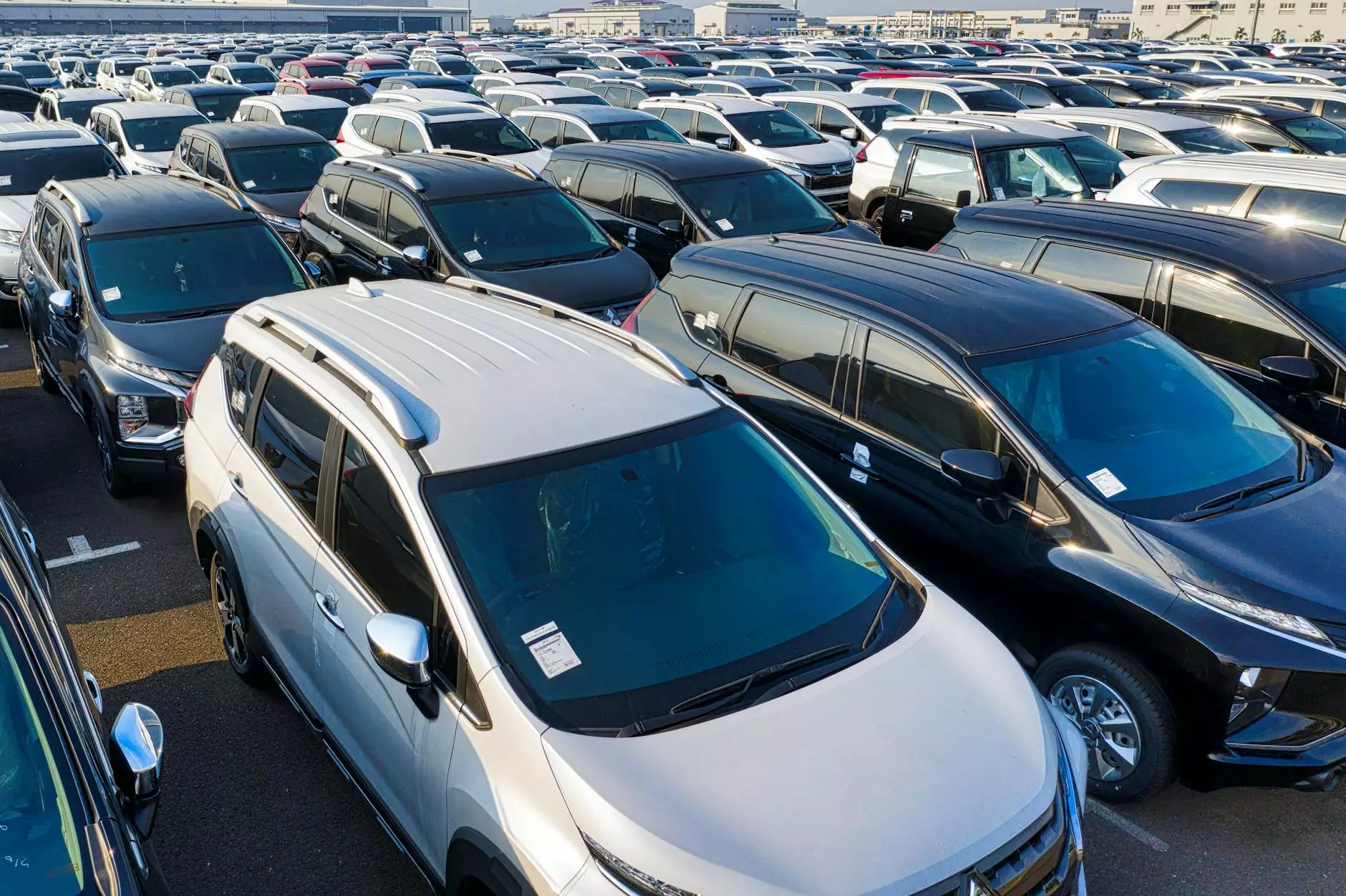
Optimize Your Rails App with Service Objects for Better Performance
As a leading SEO agency based in Buffalo, Your SEO Geek understands the importance of efficient web development practices and their impact on search engine rankings. In this article, we will delve into the concept of service objects and provide you with comprehensive guidance on refactoring your Rails app to boost its performance.
Why Refactor Your Rails App?
Before we dive into service objects, let's first understand why refactoring your Rails app is crucial. As your online presence grows, your app's functionality and complexity increase. Over time, this can lead to bloated models or controllers, making your code difficult to maintain and causing performance issues.
By refactoring your Rails app, you can modularize your code, improve its readability, and enhance overall performance. This is where service objects come into play.
What are Service Objects?
Service objects are Ruby objects that encapsulate complex business logic or repetitive processes within your Rails app. They act as intermediaries between your controllers and models, providing a structured and reusable approach to handle specific actions.
Using service objects allows you to break down your app's functionality into smaller, more manageable units. It promotes the Single Responsibility Principle (SRP) and keeps your codebase cleaner, making it easier to test, debug, and maintain.
The Benefits of Using Service Objects in Rails
Improved Code Organization: Service objects provide a logical structure for your app's business logic, keeping it separate from your controllers and models. This improves the maintainability and readability of your codebase.
Reusability: With service objects, you can encapsulate common business processes and reuse them across multiple controllers or actions. This eliminates code duplication, promoting efficiency, and reducing the chances of introducing bugs.
Enhanced Testability: Service objects are easier to test compared to models or controllers. By isolating specific business logic within a service object, you can write focused, unit tests, and ensure that each action behaves as expected.
Better Performance and Scalability: Refactoring your Rails app with service objects can lead to optimized performance. By breaking down complex processes into smaller, manageable units, you can improve response times, reduce memory consumption, and enable easier scalability as your app grows.
An Example Scenario: Implementing a User Registration Service Object
To help you understand the practical implementation of service objects, let's consider an example scenario of implementing a user registration service object in your Rails app.
Step 1: Create a User Registration Service Object
In your Rails app's app/services directory, create a new file called user_registration_service.rb. This file will contain the logic for handling user registration.
class UserRegistrationService def initialize(user_params) @user_params = user_params end def register_user // Logic for validating and saving user records end private def validate_user_params // Validate user parameters end def save_user // Save user record to the database end endStep 2: Implement User Registration in the Controller
In your app's controller, such as users_controller.rb, you can now call the UserRegistrationService to handle user registrations. By doing this, you keep your controller clean and delegate the registration logic to the service object.
def create registration_service = UserRegistrationService.new(user_params) registration_service.register_user // Handle success/failure scenarios endStep 3: Benefits and Further Enhancements
By refactoring the user registration process into a service object, you gain various benefits. It makes your code more maintainable, testable, and scalable. Moreover, you can easily reuse the UserRegistrationService in other parts of your app where user registration is required.
To further enhance your service object, you can implement error handling, email notifications, or additional validation checks to ensure a seamless user registration experience.
Consult with Buffalo's Top SEO Experts at Your SEO Geek
As reputable SEO experts based in Buffalo, Your SEO Geek understands the challenges businesses face when it comes to improving their online presence. In addition to providing valuable information on refactoring Rails apps with service objects, we offer a range of digital marketing services to optimize your website's visibility and performance.
With years of experience in the industry, our skilled team of professionals excels in delivering comprehensive SEO strategies tailored to your unique business goals. Whether you require keyword research, on-page optimization, or link building services, Your SEO Geek is here to guide you every step of the way.
Don't let your competitors outrank you. Contact Your SEO Geek, the leading SEO agency in Buffalo, and take your online presence to new heights. Our SEO consultants are ready to assist you in driving organic traffic and achieving top search engine rankings.
Conclusion
Refactoring your Rails app with service objects is a strategic approach to improving its performance and maintainability. By following the guidelines provided in this comprehensive guide, you can modularize your code, enhance reusability, and optimize your app's functionality.
Your SEO Geek, as a trusted SEO company in Buffalo, is dedicated to helping businesses succeed in the digital landscape. By combining our expertise in SEO with efficient development practices, we can assist you in outranking your competitors and achieving your online objectives.