Group Chat Room in Rails
Blog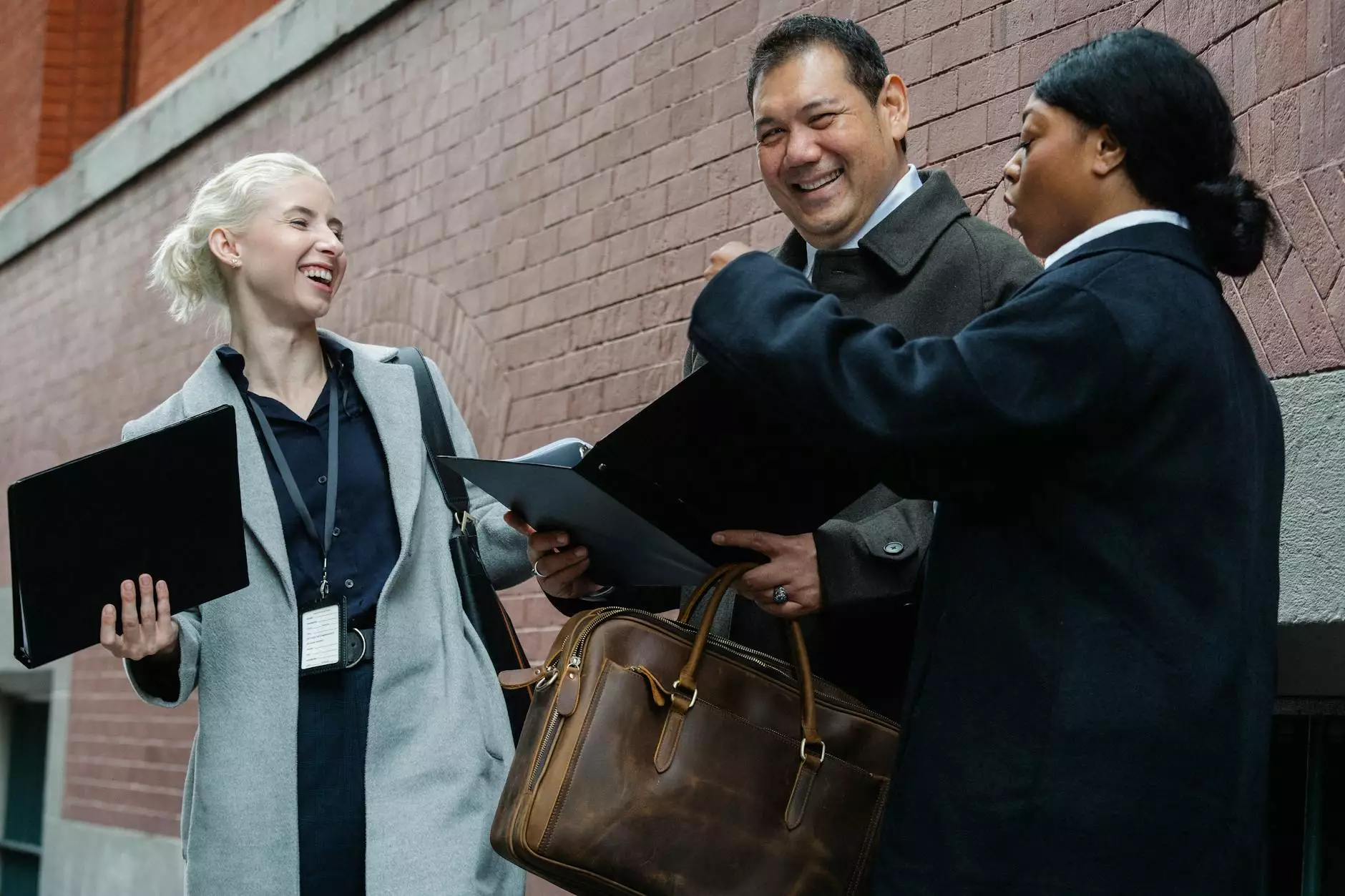
Introduction
Welcome to Your SEO Geek's article on creating a group chat room in Rails! As one of the top SEO agencies in Buffalo, we understand the importance of building engaging and interactive web applications. In this article, we will guide you through the process of setting up a group chat room in Rails, allowing you to create a real-time chat experience for your users.
Why Choose Rails?
When it comes to web development frameworks, Rails (also known as Ruby on Rails) stands out as a powerful and efficient option. With its convention-over-configuration approach, Rails allows developers to quickly build robust web applications.
By utilizing Rails for your group chat room, you gain access to its extensive ecosystem of gems and plugins, making it easier to implement features like real-time messaging and user authentication.
Setting Up the Project
To get started, make sure you have Rails installed on your machine. If not, you can visit the official Rails website and follow the installation instructions for your operating system.
Once Rails is installed, open your terminal or command prompt and navigate to your preferred directory. Run the following command to create a new Rails application:
$ rails new group_chat_roomThis will create a new Rails project named "group_chat_room". Enter the project directory with:
$ cd group_chat_roomInstalling Dependencies
Next, we need to install the necessary gems for our group chat room. Open the Gemfile in your project root directory and add the following gems:
gem 'actioncable' gem 'redis' gem 'devise'Save the file and run the following command to install the new gems:
$ bundle installCreating Models and Associations
In order to manage our chat room, we need to create some models and their associations. For simplicity, we will have two models: User and Message.
Generate the User model with the following command:
$ rails generate model User name:stringNext, generate the Message model:
$ rails generate model Message content:text user:referencesRun the database migrations:
$ rails db:migrateImplementing Real-Time Messaging - Action Cable
With Rails' integrated Action Cable framework, we can easily add real-time messaging to our chat room.
First, generate a Channel called Chat:
$ rails generate channel ChatThis will create a ChatChannel class and corresponding JavaScript file. Open app/channels/chat_channel.rb and modify it as follows:
class ChatChannel < ApplicationCable::Channel def subscribed stream_from 'chat_channel' end def receive(data) ActionCable.server.broadcast('chat_channel', message: data['message'], username: current_user.name) end endImplementing User Authentication - Devise
To provide user authentication functionality, we will integrate Devise into our application.
Add the following line to your Gemfile:
gem 'devise'Save the file and run the following command to install the Devise gem:
$ bundle installOnce the installation is complete, run the following command to set up Devise:
$ rails generate devise:installThis will create the necessary configuration files for Devise. Next, generate a User model with Devise:
$ rails generate devise UserRun the migrations:
$ rails db:migrateNow, you can add user authentication to any controller or view by simply using before_action :authenticate_user!.
Conclusion
Congratulations! You have successfully set up a group chat room in Rails. By leveraging the power of Rails, Action Cable, and Devise, you can create a highly interactive and engaging chat experience for your users.
As a leading Buffalo SEO company, Your SEO Geek recommends paying attention to user engagement and interactivity, as search engines favor websites that provide valuable and engaging content. By implementing features like group chat rooms, you can enhance user experience and improve your website's search engine rankings.
Remember, for professional SEO services in Buffalo, including expert SEO consulting, Your SEO Geek is here to help. Contact us today to boost your online presence and drive organic traffic to your website!