How to Add Functionality to Ruby Classes with Decorators
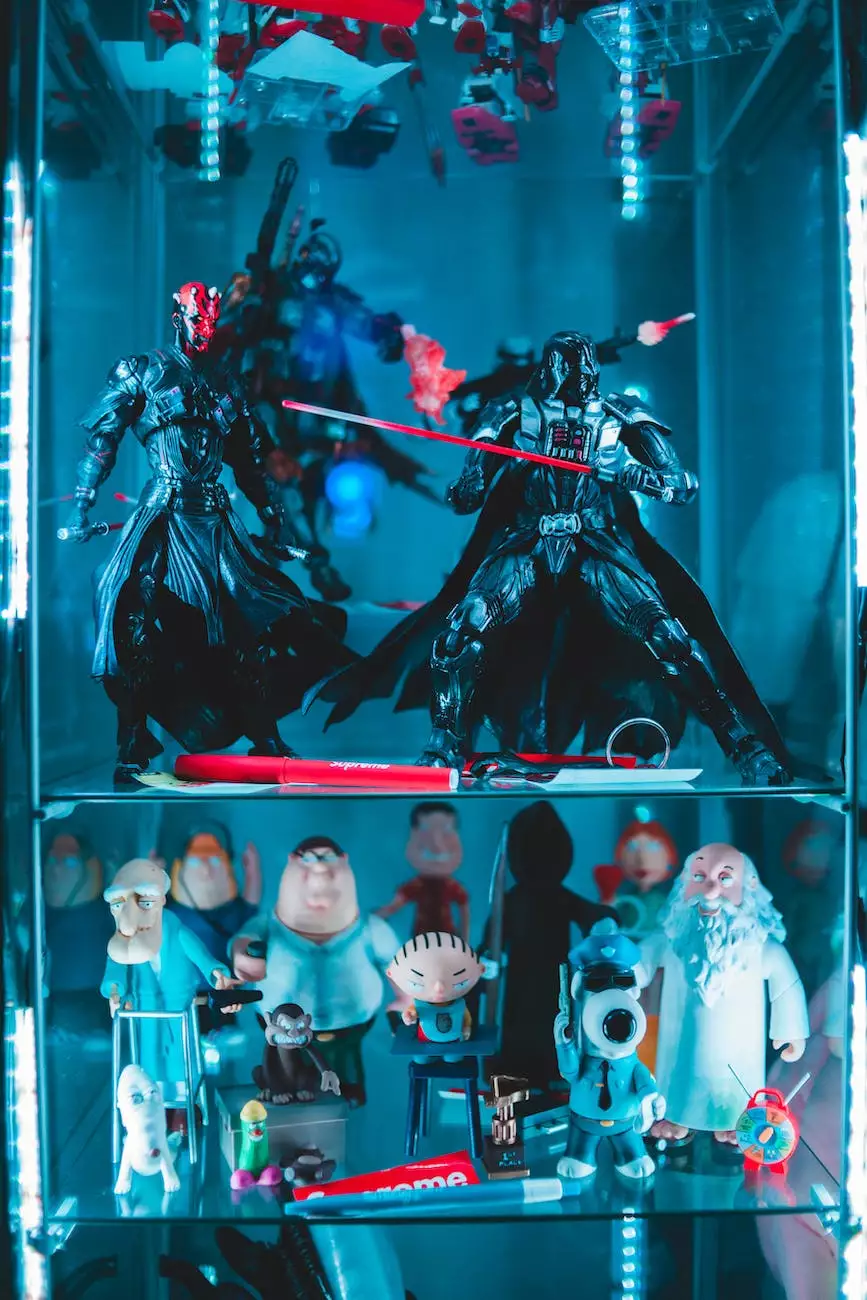
Are you a Ruby developer looking to enhance the functionality of your classes? Look no further! Your SEO Geek brings you a comprehensive guide on how to add functionality to Ruby classes with decorators, allowing you to improve the versatility and flexibility of your code. We are one of the top SEO agencies in Buffalo, specializing in helping businesses in the digital marketing industry.
Understanding Ruby Classes and Decorators
Before we dive into the details of adding functionality to Ruby classes with decorators, let's first understand the basics. Ruby classes are a fundamental building block of object-oriented programming in Ruby. They encapsulate data and behavior, allowing you to create objects with specific attributes and methods.
Decorators, on the other hand, provide a way to modify the behavior of existing classes without changing their structure. They allow you to add new features or modify existing ones dynamically. This can be especially useful when working with third-party libraries or open-source code.
The Benefits of Using Decorators
Using decorators in Ruby classes offers several advantages:
- Modularity: Decorators allow you to add or remove functionality without modifying the original class.
- Flexibility: You can apply different decorators to the same class, providing additional functionality in a modular and reusable way.
- Readability: By separating concerns into different decorators, your code becomes more organized and easier to understand.
- Code Reusability: Once you've created useful decorators, you can apply them to multiple classes, saving time and effort.
Step-by-Step Guide: Adding Functionality with Decorators
Step 1: Installing the 'decorators' Gem
Before we can start using decorators, we need to install the 'decorators' gem. Open your terminal and run the following command:
gem install decoratorsStep 2: Creating the Base Class
Let's begin by creating a basic Ruby class that we will enhance with decorators. In this example, we'll create a 'Car' class with some simple attributes and methods:
class Car attr_accessor :brand, :color, :price def initialize(brand, color, price) @brand = brand @color = color @price = price end def start_engine puts "Starting the engine of #{brand}..." # Additional logic goes here end endStep 3: Creating Decorators
Now it's time to create our decorators. Decorators are implemented as separate classes that wrap around the base class. Let's create a 'LuxuryCarDecorator' and a 'SportCarDecorator' to add specific functionalities:
require 'decorators' class LuxuryCarDecorator < SimpleDelegator def initialize(car) super(car) end def features puts "Enhancing the #{brand} with luxury features..." # Additional logic goes here end end class SportCarDecorator < SimpleDelegator def initialize(car) super(car) end def top_speed puts "Increasing the top speed of #{brand}..." # Additional logic goes here end endStep 4: Applying Decorators
Now that we have our decorators ready, let's apply them to our 'Car' class. We can do this by using the 'decorate' method provided by the 'decorators' gem:
car = Car.new("Mercedes", "Silver", 50000) decorated_car = LuxuryCarDecorator.decorate(car) decorated_car.features sporty_car = Car.new("Ferrari", "Red", 200000) sporty_car.extend(SportCarDecorator) sporty_car.top_speedStep 5: Testing the Decorated Classes
Finally, let's test our decorated classes:
car.start_engine # Output: Starting the engine of Mercedes... decorated_car.start_engine # Output: Starting the engine of Mercedes... # (additional features) sporty_car.start_engine # Output: Starting the engine of Ferrari... sporty_car.features # Output: NoMethodError: undefined method `features' for # sporty_car.top_speed # Output: Increasing the top speed of Ferrari...Conclusion
Congratulations! You've successfully learned how to add functionality to Ruby classes with decorators. By utilizing decorators, you can extend the functionality of your classes without modifying their original structure. This approach offers modularity, flexibility, and improved code readability.
At Your SEO Geek, we understand the importance of staying up-to-date with the latest trends and techniques in digital marketing. As a leading SEO company in Buffalo, we provide expert SEO services to businesses in the area. Our team of SEO experts and consultants are well-versed in improving website visibility and rankings.
Whether you're looking for SEO agencies in Buffalo, Buffalo SEO companies, or an SEO expert in Buffalo, Your SEO Geek has got you covered. Our proven strategies and comprehensive knowledge of SEO can help your website outrank the competition, driving more organic traffic and boosting your online presence.
For all your SEO needs in Buffalo and beyond, trust Your SEO Geek. Contact us today to get started on improving your website's search rankings and attracting more customers.