The Basics of Creating and using Modules in Rails - RailsCarma
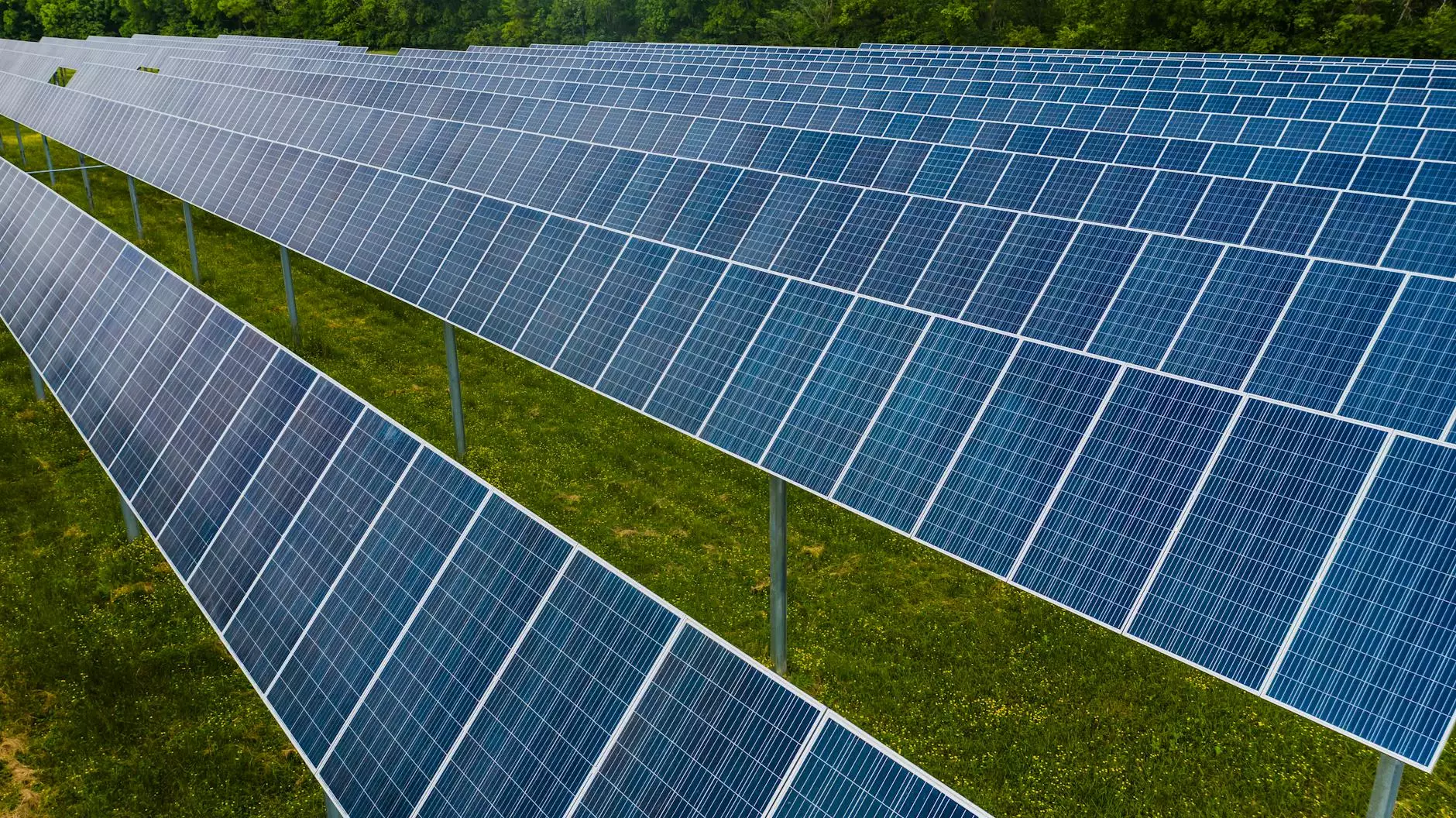
Introduction to Modules in Rails
Ruby on Rails is a powerful web development framework that allows developers to build dynamic and robust web applications. One of the key features of Rails is its support for modules. In this article, we will explore the basics of creating and using modules in Rails, and how they can enhance your development process.
What are Modules in Rails?
Modules in Rails are a way to organize related functionality into reusable units. They serve as a container for methods, constants, and other Ruby code, allowing you to encapsulate functionality and avoid conflicts with other parts of your application.
Modules can be defined inside a class or independently, and they can be included or extended in other classes or modules. This makes them extremely flexible and reusable, enabling you to share code across multiple parts of your Rails application.
Creating Modules in Rails
To create a module in Rails, you simply define it using the module keyword followed by the module's name. For example:
module MyModule # module code here endOnce you have defined a module, you can include it or extend it in other classes or modules using the include or extend keywords, respectively. This allows you to access the methods and constants defined within the module.
Using Modules in Rails
Modules are commonly used in Rails to share common functionality across models, controllers, and even views. Let's take a look at some examples of how you can use modules in different parts of your Rails application.
Using Modules in Models
In Rails, models represent the data and business logic of your application. Modules can be used to encapsulate common functionality that is shared across multiple models. For example, you can create a module that defines methods for handling image uploads and include it in multiple models that require this functionality.
module ImageUploadable def upload_image(image) # code for image upload end end class User < ApplicationRecord include ImageUploadable # other user-specific code end class Product < ApplicationRecord include ImageUploadable # other product-specific code endUsing Modules in Controllers
Controllers in Rails handle the logic behind processing requests and generating responses. Modules can be used to define common methods or filters that need to be applied to multiple controllers.
module Pagination def paginate_results # code for pagination end end class UsersController < ApplicationController include Pagination # other user-specific code end class ProductsController < ApplicationController include Pagination # other product-specific code endUsing Modules in Views
Views in Rails are responsible for rendering the user interface. Modules can be used to define helper methods or provide shared functionality for views.
module FormattingHelper def format_date(date) # code for date formatting end end class UsersController < ApplicationController include FormattingHelper # other user-specific code end class ProductsController < ApplicationController include FormattingHelper # other product-specific code endConclusion
In conclusion, modules provide a powerful way to organize and reuse code in your Rails application. By using modules, you can encapsulate functionality, avoid conflicts, and make your code more maintainable and scalable. Whether you need to share functionality across models, controllers, or views, modules can help you achieve cleaner and more efficient code.
If you are looking for SEO agencies in Buffalo, look no further than Your SEO Geek. As a top-rated SEO company in Buffalo, we have the expertise and experience to help your website rank higher in search engine results. Our SEO experts in Buffalo are skilled in optimizing websites for search engines, ensuring maximum visibility and organic traffic. Contact our Buffalo SEO consultant today to discuss your SEO needs and take your online presence to the next level!