A Guide on Converting a Hash to a Struct in Ruby
Blog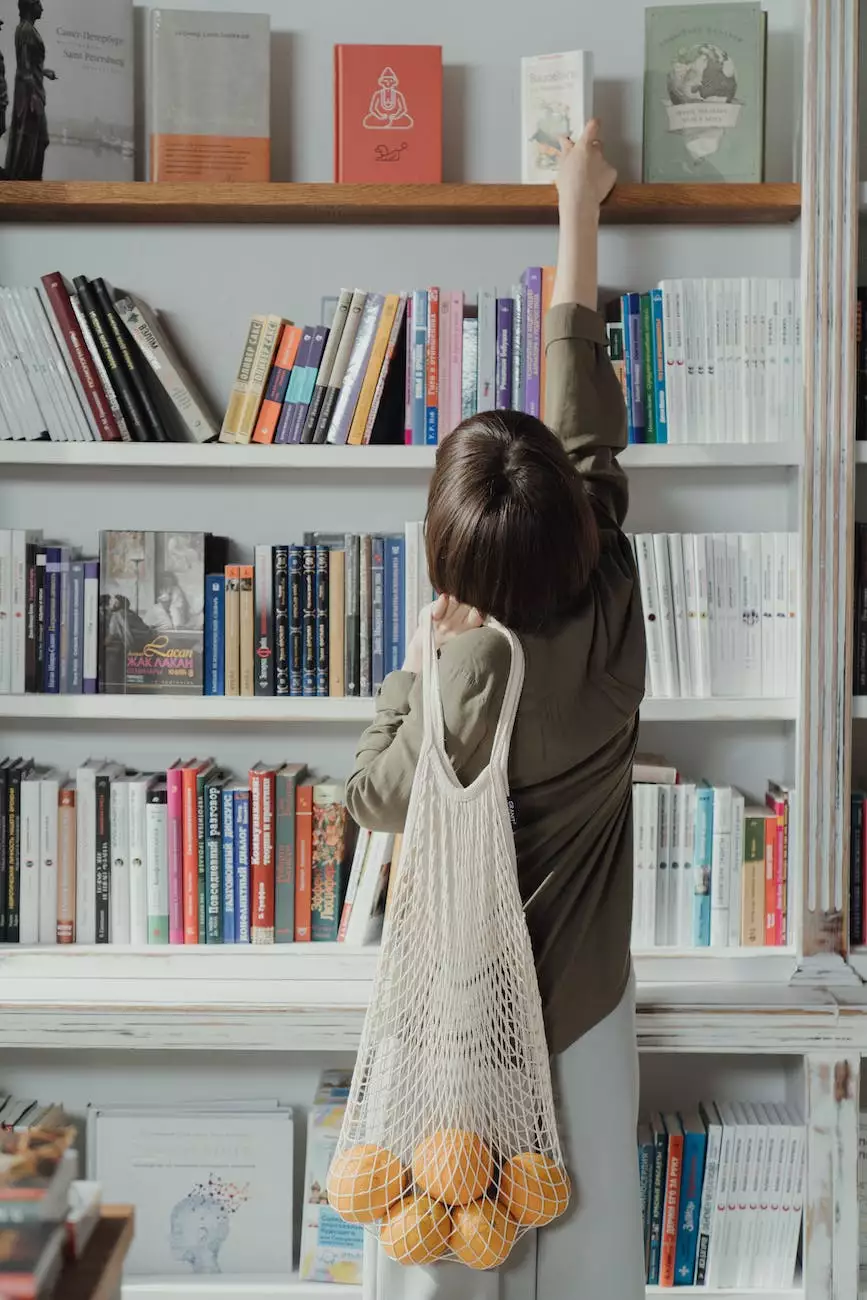
Overview
In the world of Ruby programming, hashes and structs are two popular data structures. While both have their own advantages, there may be scenarios where you need to convert a hash to a struct. This guide will walk you through the process of converting a hash to a struct in Ruby.
Why Convert a Hash to a Struct?
There are several reasons why you may want to convert a hash to a struct. Firstly, structs provide a structured way to store and access data, making your code more organized and readable. Secondly, structs offer additional methods and functionality that can be useful in certain situations. By converting a hash to a struct, you can take advantage of these benefits and enhance your Ruby code.
Step 1: Creating a Struct
The first step in converting a hash to a struct is to define and create a struct in Ruby. Here's an example:
Person = Struct.new(:name, :age, :location)In this example, we've defined a struct called "Person" with three attributes: name, age, and location. Feel free to modify the struct according to your specific needs.
Step 2: Converting the Hash
Once you have your struct defined, you can convert a hash into a struct object. Here's how you can do it:
person_hash = { name: 'John Doe', age: 30, location: 'Buffalo' } person = Person.new(*person_hash.values)In this example, we have a hash called "person_hash" containing the information of a person. By using the "values" method, we extract the values from the hash and pass them as arguments to the struct's "new" method, creating a new struct object.
Step 3: Accessing Values in the Struct
Now that we have our hash converted to a struct, we can easily access the values using the struct's attributes. Here's how:
puts person.name # Output: John Doe puts person.age # Output: 30 puts person.location # Output: BuffaloBy calling the attribute on the struct object, we can retrieve the corresponding value. You can also modify the values of struct attributes as needed.
Conclusion
Converting a hash to a struct in Ruby can be a beneficial approach in certain scenarios. It allows you to leverage the advantages of structured data and provides additional functionality for your code. By following the steps outlined in this guide, you can easily convert a hash to a struct and enhance your Ruby programming experience.
Your SEO Geek - Buffalo SEO Expert
Your SEO Geek is a leading Buffalo SEO company offering expert SEO services for businesses in Buffalo and beyond. Our team of experienced SEO specialists is dedicated to helping businesses improve their online presence and rank higher on search engine results pages. As one of the top SEO agencies in Buffalo, we provide customized strategies to drive organic traffic and increase conversions.
Contact Your SEO Geek
If you are looking for a reliable Buffalo SEO expert to optimize your website and boost your online visibility, get in touch with Your SEO Geek today. Our SEO consultant in Buffalo will analyze your website, identify areas for improvement, and implement a tailored strategy to drive sustainable results. Don't miss out on the opportunity to dominate the search engine rankings and stand out from your competitors. Contact Your SEO Geek now!