How to Achieve Parallelism with Ruby MRI with I/O Bound Threads
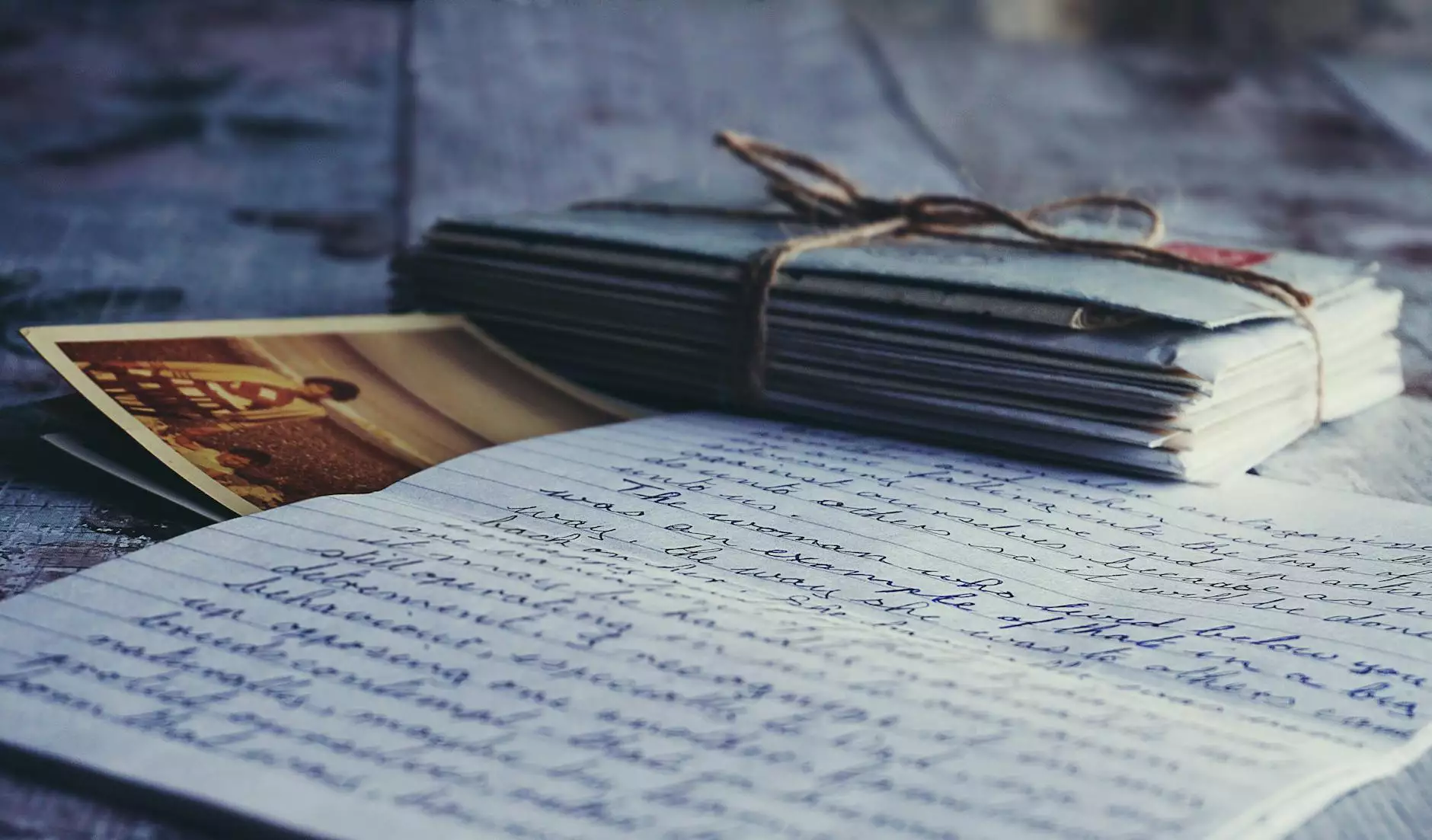
Introduction
Ruby MRI (Matz's Ruby Interpreter) is a popular implementation of the Ruby programming language, widely used for its simplicity and expressiveness. However, when dealing with I/O bound operations, such as database queries or network requests, Ruby MRI's performance can be limited due to its lack of inherent parallelism.
The Challenge of Parallelism with Ruby MRI
Parallelism refers to the ability of a program to execute multiple tasks simultaneously. This can have a significant impact on performance, especially when dealing with I/O bound operations that would otherwise cause delays.
In the context of Ruby MRI, achieving parallelism can be challenging due to its Global Interpreter Lock (GIL). The GIL restricts Ruby MRI from executing multiple threads in parallel, limiting the performance gains that could be achieved through parallel execution of I/O bound tasks.
The Solution: Leveraging Concurrent Ruby
To overcome the limitations of Ruby MRI, we can leverage the power of Concurrent Ruby, a gem that provides high-level concurrency abstractions and tools.
Step 1: Installing Concurrent Ruby
To get started, you need to install Concurrent Ruby gem using the following command:
gem install concurrent-rubyStep 2: Implementing Parallelism with Concurrent Ruby
Concurrent Ruby offers several constructs that facilitate parallelism. One of the most commonly used constructs is the `ThreadPoolExecutor`, which allows you to define a pool of threads that can execute tasks concurrently.
Here's an example of how you can use `ThreadPoolExecutor` to achieve parallelism in Ruby MRI:
require 'concurrent' # Create a new thread pool with a maximum concurrency of 10 thread_pool = Concurrent::ThreadPoolExecutor.new(max_threads: 10) # Submit I/O bound tasks to the thread pool thread_pool.post do # Your I/O bound task 1 end thread_pool.post do # Your I/O bound task 2 end # Wait for all tasks to complete thread_pool.shutdown thread_pool.wait_for_terminationStep 3: Optimizing Parallel Execution
While Concurrent Ruby provides the necessary tools for parallelism, achieving optimal performance requires careful consideration of various factors. Here are some tips to optimize the parallel execution of I/O bound tasks:
1. Task Scheduling
Decide on the optimal number of threads in your thread pool based on the nature of your tasks and the available system resources. Experimentation and benchmarking can guide you in finding the right balance.
2. Chunking
If you have a large number of similar I/O bound tasks, consider dividing them into chunks and distributing them across multiple threads. This can prevent bottlenecks and improve overall parallel performance.
3. Load Balancing
Avoid thread starvation by ensuring an equal distribution of tasks among threads. Load balancing techniques, such as work stealing or task stealing, can help achieve better parallel performance.
Conclusion
With the combination of Ruby MRI and Concurrent Ruby, achieving parallelism in I/O bound operations is within reach. By following the steps outlined in this guide and optimizing the parallel execution, you can unlock the full potential of your Ruby applications and improve overall performance.
As a leading Buffalo SEO company, Your SEO Geek is dedicated to helping businesses achieve top rankings in search engines. Our expert Buffalo SEO consultants have the knowledge and expertise to optimize your website and drive quality organic traffic. Contact us now to take your digital marketing strategy to new heights!